Site survey apps
This tutorial shows how to integrate your site survey app with Aurora.
Private beta
- Beta product features will not be supported via our normal support channels (e.g., email).
- You may experience some gaps or changes as the product is refined and documentation updated.
- There may be some compatibility issues between our beta and generally available product.
How It Works
When a mutual customer requests a site survey, Aurora will notify your app of the request along with some information about the site. After the survey is complete, your apps publishes results using Aurora Sync API.
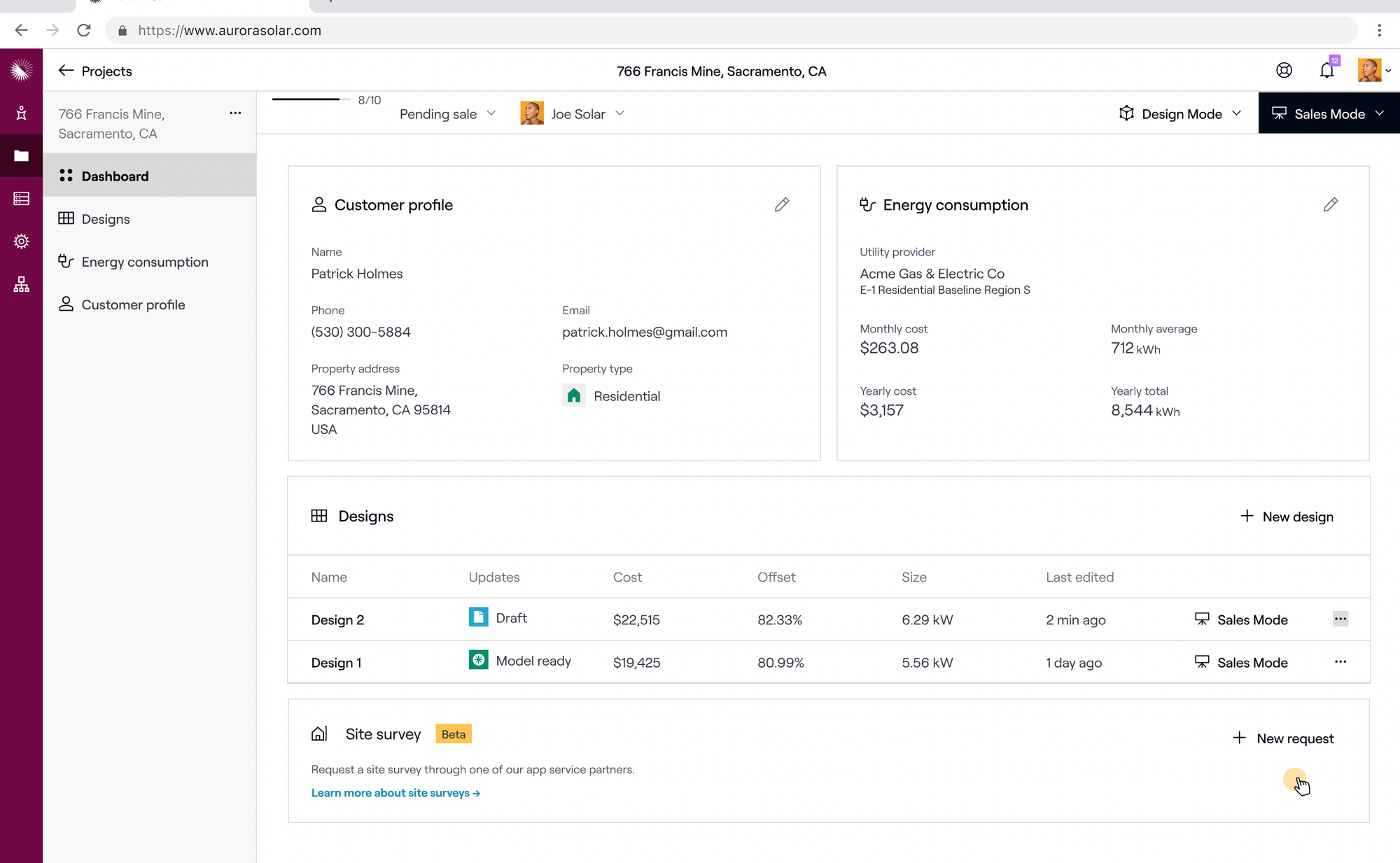
Users can request a site survey in Aurora app
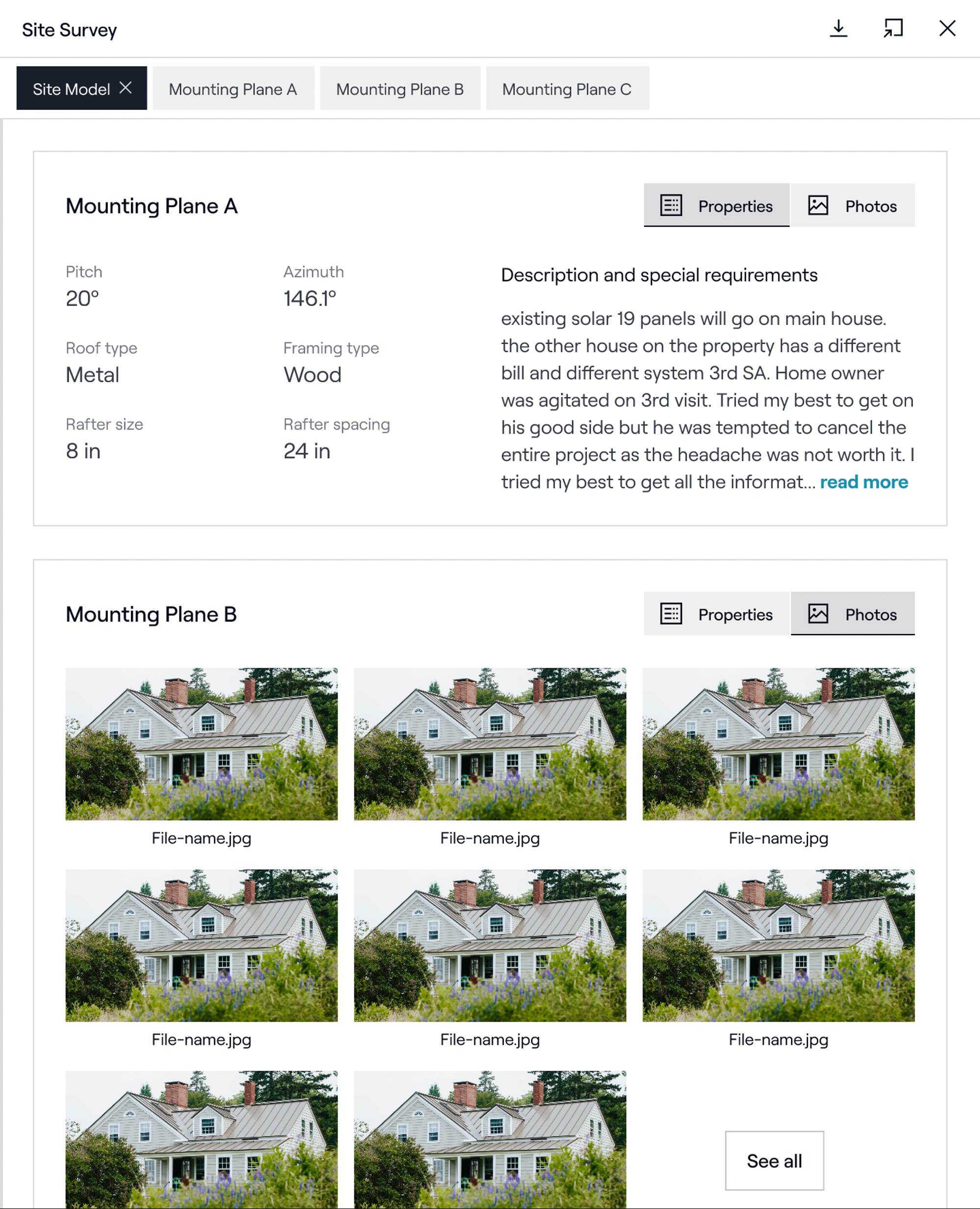
After you upload site survey results, users can review them in Aurora app
One-Time Setup
Provide your Aurora account team with:
- your Aurora sandbox
tenant_id
, - your company name as you want users to see in Aurora UI,
- your company logos as you want users to see in Aurora UI,
- one-sentence customer-facing description of your app,
Aurora account team will notify you when your tenant setup is complete, providing you with the application key and application_id
.
Step 1. Subscribe to site_survey_requested partner webhook event.
Subscribe to site_survey_requested
partner webhook event, pointing webhooks to your application that will process them. See Webhooks for more details.
When an Aurora user requests a site survey, Aurora will send you a webhook with the following payload:
//Webhook query string payload
?site_survey_request_id=<SITE_SURVEY_REQUEST_ID>&tenant_id=<TENANT_ID>&session_token=<SESSION_TOKEN>
Step 2. Retrieve temporary key
Using the aurora_session_token
value from the query parameters, your application_id
and your application key provided by Aurora, make a request to generate a new temporary key. To avoid exposing your application key, this request should always happen server-side.
curl -X POST \
-H "Authorization: Bearer {your application key}" \
"https://api-sandbox.aurorasolar.com/partner_applications/{application_id}/tenants/{aurora_tenant_id}/create_token?session_token={aurora_session_token}"
{
"token": {
"bearer_token": "tk_test_fd1cc8562360ff06b6e524aa",
"expires_at": "2023-07-29 04:30:46 UTC"
}
}
Please note that site survey applications are considered backend apps and do not require the session_token
parameter when creating temporary tokens. This allows you to generate a token when there’s a long delay between receiving a site_survey_requested
webhook and the survey being performed. However we recommend including the session_token
as much as possible to improve security for our shared customers. For example, if you need to look up project data immediately after receiving the webhook, we strongly encourage you to include it.
Step 3. Retrieve site survey request and project information
Using the temporary key and the Aurora Sync API, you can retrieve information about the site survey request, the site, and the proposed design.
The Retrieve Site Survey Request endpoint will give you the project and design IDs that the site survey was requested for, as well as a URL to a screenshot that the Aurora customer took of the design.
//GET <https://api-sandbox.aurorasolar.com/tenants/{tenant_id}/site_surveys/{site_survey_request_id}/request
//HTTP 200 response
{
"site_survey_request": {
"id": "3fa85f64-5717-4562-b3fc-2c963f66afa6",
"project_id": "3fa85f64-5717-4562-b3fc-2c963f66afa6",
"requested_for_design_id": "3fa85f64-5717-4562-b3fc-2c963f66afa6",
"status": "requested",
"requested_at": "2024-01-25T07:21:30.507Z",
"completed_at": null,
"screenshot_url": "www.imagehost.com/file1.png"
}
}
The Retrieve Project endpoint will give you information about the project site and owner.
//GET <https://api-sandbox.aurorasolar.com/tenants/{tenant_id}/projects/{project_id}
//HTTP 200 response
{
"project": {
"customer_salutation": "Mrs.",
"customer_first_name": "Jane",
"customer_last_name": "Doe",
"customer_address": "434 Brannan St, San Francisco, CA, USA",
"customer_email": "[email protected]",
"customer_phone": "(555) 111-5151",
"owner_id": "3fa85f64-5717-4562-b3fc-2c963f66afa6",
"team_id": "3fa85f64-5717-4562-b3fc-2c963f66afa6",
"address": "434 Brannan St, San Francisco, CA, 94107",
"latitude": 37.77960043,
"longitude": -122.39530086,
"project_type": "residential",
"ahj_id": "3fa85f64-5717-4562-b3fc-2c963f66afa6",
"created_at": "2024-01-25T18:35:07.345Z",
"updated_at": "2024-01-25T18:35:07.345Z",
...
}
}
The retrieve Design Summary endpoint will give you information about the batteries .
//GET <https://api-sandbox.aurorasolar.com/tenants/{tenant_id}/designs/{design_id}/summary
//HTTP 200 response
{
"design": {
"bill_of_materials": [
{
"id": "3fa85f64-5717-4562-b3fc-2c963f66afa6",
"component_type": "batteries",
"sku": null,
"name": "Aurora Sample Battery",
"manufacturer_name": "Aurora Sample Manufacturer",
"quantity": 2
},
...
]
}
}
The full list of Sync API endpoints you have access to is determined by the following access scopes:
read_projects
read_designs
read_site_surveys
write_site_surveys
Step 4. Update the site survey
After completing the site survey, upload the results to Aurora using the suite of Site Survey Update Sync APIs.
-
Upload electrical data using Update Site Survey Electrical Data.
// PUT <https://api-sandbox.aurorasolar.com/tenants/{tenant_id}/site_surveys/{site_survey_request_id}/electrical // Request body { "electrical": { "main_panel_location": "<string>", // required at completion (enum) "main_bus_rating": "<float>", // amperes, required at completion "main_breaker_rating": "<float>", // amperes, required at completion "service_type": "<string>", // (enum) "feeder_type": "<string>", // (enum) "utility_meter_location": "<string>", // (enum) "existing_grounding": "<string>", // (enum) "existing_grounding_location": "<string>", "main_panel_manufacturer": "<string>", "notes": "<string>", "images": [ { "url": "https://example.com/image.jpg", // public URL for an image. Aurora will "filename": "image.jpg" // download and save images from given URL }, ] } }
-
Upload subpanels data using Update Site Survey Subpanels.
// PUT <https://api-sandbox.aurorasolar.com/tenants/{tenant_id}/site_surveys/{site_survey_request_id}/electrical/subpanels // Request body { "subpanels": [ { "location": "<string>", "disconnect_location": "<string>", "bus_rating": "<integer>", // amperes "disconnect_size": "<integer>", // amperes, "notes": "<string>", "images": [ { "url": "https://example.com/image.jpg", // public URL for an image. Aurora will "filename": "image.jpg" // download and save images from given URL }, ] } ] }
-
Upload mounting planes data using Update Mounting Planes.
// PUT <https://api-sandbox.aurorasolar.com/tenants/{tenant_id}/site_surveys/{site_survey_request_id}/roofs/mounting_planes // Request body { "mounting_planes": [ { "units_of_measurement": "<string>", // optional "pitch": "<float>", // required "azimuth": "<float>", // required "roof_type": "<string>", // required (enum) "framing_type": "<string>", // required (enum) "rafter_size": "<string>", // required (enum) "rafter_spacing": "<integer>", //required (inches or m) "notes": "<string>", "images": [ { "url": "https://example.com/image.jpg", // public URL for an image. Aurora will "filename": "image.jpg" // download and save images from given URL }, ] } ] }
Step 5. Mark the site survey request as “completed”
To notify the customer that the site survey is completed, call the Update Site Survey Status endpoint and set the status to completed
.
// PUT <https://api-sandbox.aurorasolar.com/tenants/{tenant_id}/site_surveys/{site_survey_request_id}/status
// Request body
{
"site_survey": {
"status": "completed"
}
}
This endpoint will also do validation and return a HTTP 422 with a list of errors if the Site Survey fields marked “required at completion” are not provided.
Considerations
- All endpoints and the webhook mentioned in this guide are available today with the exception of the following four endpoints. The following endpoint and supporting documentation are expected to become available in early Feb '24.
- Update Site Survey Status
- The URL returned by Retrieve Site Survey Request API expires after 24 hours.
- Consider using a webhook test site like https://webhook.site to verify that your webhook subscription is live.
Updated 7 months ago